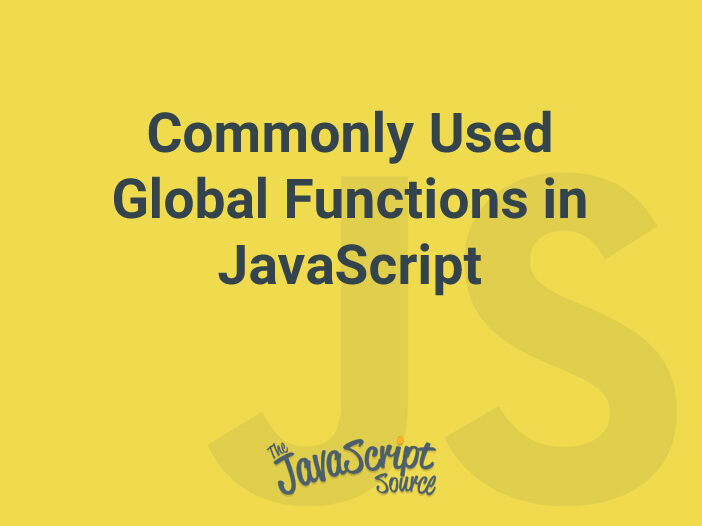
JavaScript has several built-in global functions that can be used in any JavaScript code, regardless of where the code is located. These functions are available on the global object, which is the highest level object in the JavaScript environment. In this article, we will discuss some of the most commonly used global functions in JavaScript and provide code examples for each.
parseInt()
This function is used to convert a string or a number to an integer. It takes two arguments, the first being the value to be converted, and the second being the radix (base) of the number system. The radix is optional and defaults to 10.
var num1 = parseInt("123"); // 123
var num2 = parseInt("11", 2); // 3
parseFloat()
This function is similar to parseInt(), but it is used to convert a string or a number to a floating-point number.
var num1 = parseFloat("3.14"); // 3.14
var num2 = parseFloat("11.11"); // 11.11
isNaN()
This function is used to check if a value is NaN (Not a Number). It returns true if the value is NaN, and false otherwise.
var num1 = NaN;
console.log(isNaN(num1)); // true
var num2 = "Hello";
console.log(isNaN(num2)); // true
isFinite()
This function is used to check if a value is a finite number. It returns true if the value is a finite number, and false otherwise.
var num1 = Infinity;
console.log(isFinite(num1)); // false
var num2 = 123;
console.log(isFinite(num2)); // true
decodeURI()
This function is used to decode an encoded URI (Uniform Resource Identifier). It replaces all encoded characters with their corresponding unencoded characters.
var uri = "https://www.example.com?name=John%20Doe";
console.log(decodeURI(uri)); // "https://www.example.com?name=John Doe"
encodeURI()
This function is used to encode a URI. It replaces all special characters with their corresponding encoded characters.
var uri = "https://www.example.com?name=John Doe";
console.log(encodeURI(uri)); // "https://www.example.com?name=John%20Doe"
eval()
This function is used to execute a string of JavaScript code. It should be used with caution as it can open up security vulnerabilities if not used properly.
var code = "console.log('Hello World!')";
eval(code); // Output: "Hello World!"
escape()
This function is used to encode a string. It replaces certain characters with their corresponding escape sequences.
var str = "Hello World!";
console.log(escape(str)); // "Hello%20World%21"
unescape()
This function is used to decode an encoded string. It replaces all escape sequences with their corresponding characters.
var str = "Hello%20World%21";
console.log(unescape(str)); // "Hello World!"
Number()
This function is used to convert a value to a number. It can be used to convert a string, boolean, or object to a number.
var num1 = Number("123"); // 123
var num2 = Number(true); // 1
var num3 = Number({}); // NaN
Boolean()
This function is used to convert a value to a boolean. It can be used to convert a string, number, or object to a boolean.
var bool1 = Boolean("hello"); // true
var bool2 = Boolean(0); // false
var bool3 = Boolean({}); // true
String()
This function is used to convert a value to a string. It can be used to convert a number, boolean, or object to a string.
var str1 = String(123); // "123"
var str2 = String(true); // "true"
var str3 = String({}); // "[object Object]"
These are some of the most commonly used global functions in JavaScript. These functions provide a way to perform various operations on data, such as converting data types, encoding and decoding strings, and evaluating code. It’s important to keep in mind that while these functions can be very useful, they should be used with caution as they can open up security vulnerabilities if not used properly.