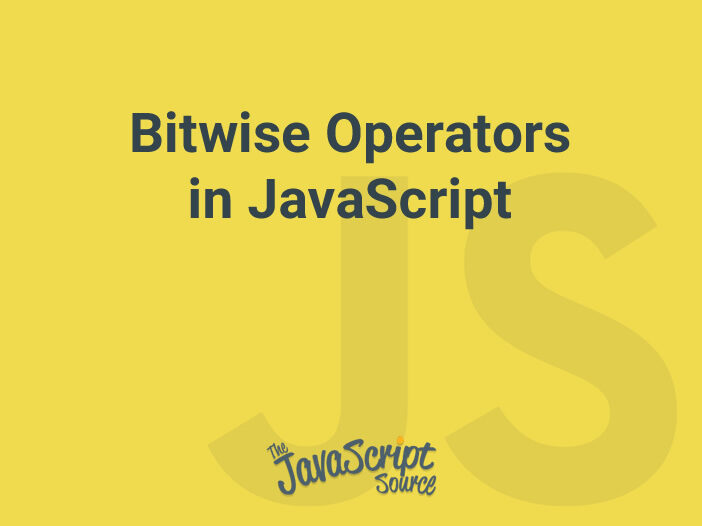
JavaScript provides several bitwise operators that allow you to manipulate the individual bits within a value. These operators include:
- Bitwise AND (
&
) - Bitwise OR (
|
) - Bitwise XOR (
^
) - Bitwise NOT (
~
) - Left shift (
<<
) - Right shift (
>>
) - Zero-fill right shift (
>>>
)
Here’s a brief explanation of each operator and an example of how to use it:
Bitwise AND (&)
The bitwise AND operator compares each bit of the first operand to the corresponding bit of the second operand. If both bits are 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
let a = 5; // 101
let b = 3; // 011
let c = a & b; // 001 = 1
In this example the a and b are binary representation of 5 and 3 respectively and c will be 1.
Bitwise OR (|)
The bitwise OR operator compares each bit of the first operand to the corresponding bit of the second operand. If either bit is 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
let a = 5; // 101
let b = 3; // 011
let c = a | b; // 111 = 7
In this example the a and b are binary representation of 5 and 3 respectively and c will be 7.
Bitwise XOR (^)
The bitwise XOR operator compares each bit of the first operand to the corresponding bit of the second operand. If the bits are different, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
let a = 5; // 101
let b = 3; // 011
let c = a ^ b; // 110 = 6
In this example the a and b are binary representation of 5 and 3 respectively and c will be 6.
Bitwise NOT (~)
The bitwise NOT operator inverts the bits of its operand. Each 0 bit is set to 1, and each 1 bit is set to 0.
let a = 5; // 101
let b = ~a; // 010 = 2
In this example the a is binary representation of 5 and b will be 2.
Left shift (<<)
The left shift operator shifts the bits of its first operand to the left by the number of positions specified by its second operand. Each shift to the left is equivalent to multiplication by 2.
let a = 5; // 101
let b = a << 2; // 10100 = 20
In this example the a is binary representation of 5 and b will be 20.
Right shift (>>)
The right shift operator shifts the bits of its first operand to the right by the number of positions specified by its second operand. Each shift to the right is equivalent to division by 2.
let a = 5; // 101
let b = a >> 2; // 001 = 1
In this example the a is binary representation of 5 and b will be 1.
Zero-fill right shift (>>>)
The zero-fill right shift operator is similar to the right shift operator, but it always fills the leftmost bits with 0s, regardless of the sign of the original value. This can be useful when working with unsigned integers.
let a = -5; // 1111111111111111111111111111011
let b = a >>> 2; // 0011111111111111111111111111101 = 1073741821
In this example the a is binary representation of -5 and b will be 1073741821.
In conclusion, JavaScript provides several bitwise operators that allow you to manipulate the individual bits within a value. These operators include: Bitwise AND (&
), Bitwise OR (|
), Bitwise XOR (^
), Bitwise NOT (~
), Left shift (<<
), Right shift (>>
) and Zero-fill right shift (>>>
). Each operator has its own specific use case and it is important to understand when and how to use them for optimal performance and code efficiency.