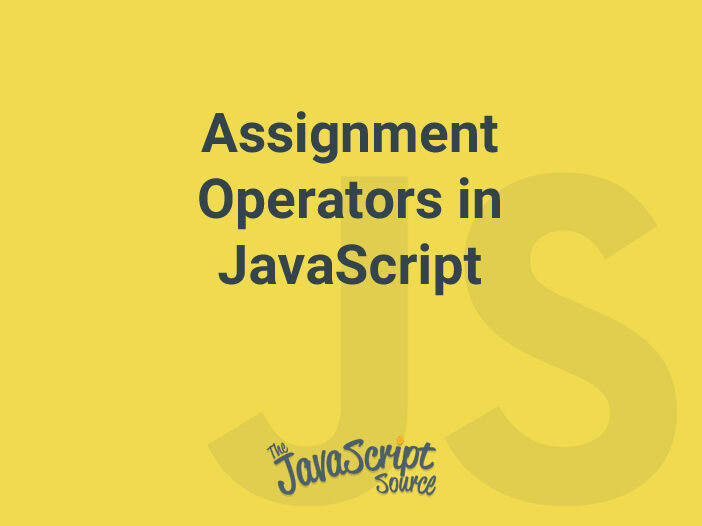
JavaScript has several assignment operators that can be used to assign values to variables. These operators are as follows:
The “=” operator: This is the most basic assignment operator and is used to assign a value to a variable. For example:
let x = 5;
The “+=” operator: This operator is used to add the value on the right side of the operator to the value of the variable on the left side, and then assign the result to the variable on the left side. For example:
let x = 5;
x += 3; // x is now 8
The “-=” operator: This operator is used to subtract the value on the right side of the operator from the value of the variable on the left side, and then assign the result to the variable on the left side. For example:
let x = 5;
x -= 3; // x is now 2
The “*=” operator: This operator is used to multiply the value on the right side of the operator by the value of the variable on the left side, and then assign the result to the variable on the left side. For example:
let x = 5;
x *= 3; // x is now 15
The “/=” operator: This operator is used to divide the value of the variable on the left side by the value on the right side of the operator, and then assign the result to the variable on the left side. For example:
let x = 15;
x /= 3; // x is now 5
The “%=” operator: This operator is used to divide the value of the variable on the left side by the value on the right side of the operator, and then assigns the remainder to the variable on the left side. For example:
let x = 15;
x %= 3; // x is now 0
The “**=” operator: This operator is used to raise the value of the variable on the left side by the power of the value on the right side of the operator.
let x = 2;
x **= 3; // x is now 8
These operators are a shorthand way to perform an operation and assign the result to a variable in a single step, which can make your code more concise and readable.