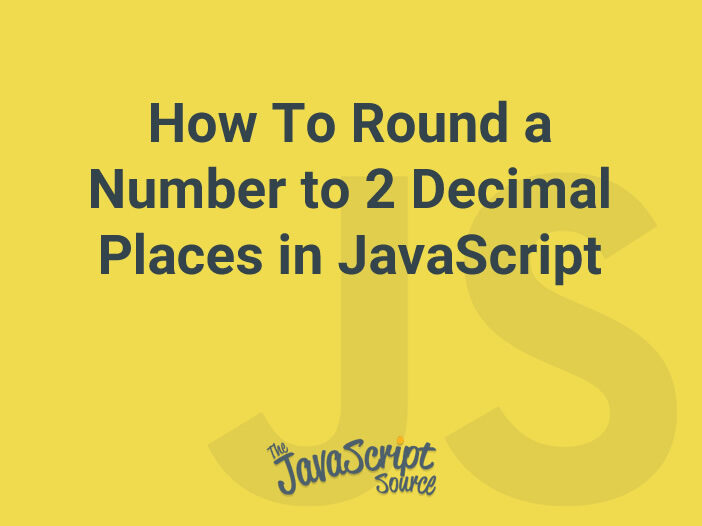
Rounding a number to a specific decimal place in JavaScript can be accomplished using the .toFixed()
method. This method rounds a number to the number of decimal places specified, and returns the result as a string.
Here is an example of using the .toFixed()
method to round a number to 2 decimal places:
var number = 3.14159;
var roundedNumber = number.toFixed(2);
console.log(roundedNumber); // Output: "3.14"
In this example, the variable number
is set to the value of 3.14159, and the .toFixed(2)
method is used to round this number to 2 decimal places. The result, 3.14, is then assigned to the variable roundedNumber
, which is then logged to the console.
It’s important to note that the .toFixed()
method returns a string, not a number. If you need to work with the rounded number as a number, you can use the parseFloat()
method to convert the string back to a number:
var number = 3.14159;
var roundedNumber = parseFloat(number.toFixed(2));
console.log(roundedNumber); // Output: 3.14
console.log(typeof roundedNumber); // Output: "number"
Another way to round a number to 2 decimal places, is by using the Math.round()
method. This method rounds a number to the nearest integer. We can use this method and divide the number by 100 and then multiply by 100.
var number = 3.14159;
var roundedNumber = Math.round(number * 100) / 100;
console.log(roundedNumber); // Output: 3.14
In this example, we first multiply the number by 100, then round it to the nearest integer, and finally divide it by 100. This will result in a number rounded to 2 decimal places.