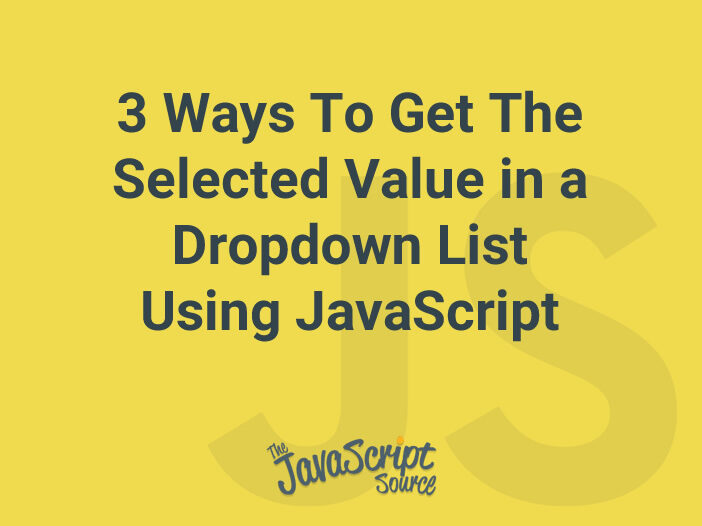
JavaScript provides several methods for getting the selected value in a dropdown list. In this article, we’ll discuss three of the most common methods: using the value
property, using the selectedIndex
property, and using the options
collection.
Method 1: Using the value
Property
The value
property of a select element returns the value of the selected option. The following example demonstrates how to use the value
property to get the selected value in a dropdown list:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Dropdown Example</title>
</head>
<body>
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
<button onclick="getSelectedValue()">Get Selected Value</button>
<script>
function getSelectedValue() {
var select = document.getElementById("mySelect");
var selectedValue = select.value;
alert(selectedValue);
}
</script>
</body>
</html>
In this example, we have a dropdown list with the id “mySelect” and three options with values “option1”, “option2”, and “option3”. When the button is clicked, the getSelectedValue()
function is called. The function gets a reference to the select element using the getElementById()
method, and assigns the value of the selected option to the variable selectedValue
. An alert box is then used to display the selected value.
Method 2: Using the selectedIndex
Property
The selectedIndex
property of a select element returns the index of the selected option. The following example demonstrates how to use the selectedIndex
property to get the selected value in a dropdown list:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Dropdown Example</title>
</head>
<body>
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
<button onclick="getSelectedValue()">Get Selected Value</button>
<script>
function getSelectedValue() {
var select = document.getElementById("mySelect");
var selectedIndex = select.selectedIndex;
var options = select.options;
var selectedValue = options[selectedIndex].value;
alert(selectedValue);
}
</script>
</body>
</html>
In this example, we again have a dropdown list with the id “mySelect” and three options with values “option1”, “option2”, and “option3”. When the button is clicked, the getSelectedValue()
function is called. The function gets a reference to the select element using the getElementById()
method, and assigns the selected index to the variable selectedIndex
. It then gets a reference to the options collection and assigns the value of the selected option to the variable selectedValue
.
Method 3: Using the options
Collection
The options
property of a select element returns a collection of all the option elements in the dropdown list. We can use this collection to iterate through all the options and find the selected one. The following example demonstrates how to use the options
collection to get the selected value in a dropdown list:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Dropdown Example</title>
</head>
<body>
<select id="mySelect">
<option value="option1">Option 1</option>
<option value="option2">Option 2</option>
<option value="option3">Option 3</option>
</select>
<button onclick="getSelectedValue()">Get Selected Value</button>
<script>
function getSelectedValue() {
var select = document.getElementById("mySelect");
var options = select.options;
var selectedValue;
for (var i = 0; i < options.length; i++) {
if (options[i].selected) {
selectedValue = options[i].value;
break;
}
}
alert(selectedValue);
}
</script>
</body>
</html>
In this example, we again have a dropdown list with the id “mySelect” and three options with values “option1”, “option2”, and “option3”. When the button is clicked, the getSelectedValue()
function is called. The function gets a reference to the select element using the getElementById()
method, and assigns the options collection to the variable options
. It then uses a for loop to iterate through all the options and check the selected
property of each option. If the property is true, it assigns the value of the option to the variable selectedValue
and breaks out of the loop. An alert box is then used to display the selected value.