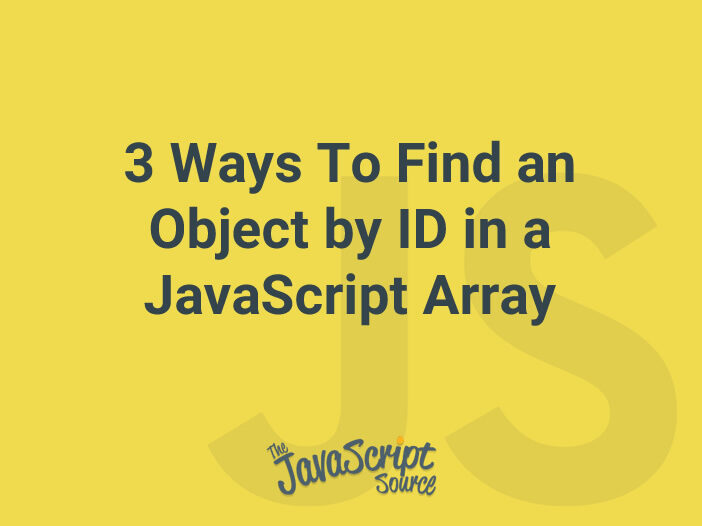
Finding an object by its ID in a JavaScript array can be a common task when working with data in your application. There are several ways to accomplish this, and in this article, we will explore three of the most popular methods.
The Array.prototype.find()
method
This method iterates over the array and returns the first element that satisfies the provided testing function. For example, if you have an array of objects like this:
const users = [
{id: 1, name: 'John'},
{id: 2, name: 'Jane'},
{id: 3, name: 'Bob'}
];
You can use the find()
method to find an object with a specific ID like this:
const user = users.find(user => user.id === 2);
console.log(user); // {id: 2, name: 'Jane'}
The Array.prototype.filter()
method
This method creates a new array with all elements that pass the test implemented by the provided function. For example, you can use filter()
to find an object with a specific ID like this:
const user = users.filter(user => user.id === 2)[0];
console.log(user); // {id: 2, name: 'Jane'}
The Array.prototype.forEach()
method
This method iterates over the array and applies the provided function for each element. For example, you can use forEach()
to find an object with a specific ID like this:
let user;
users.forEach(function(item) {
if (item.id === 2) {
user = item;
}
});
console.log(user); // {id: 2, name: 'Jane'}